Setting Up a PHP Development Environment
Getting started with PHP development can seem like a daunting task, but it doesn't have to be. Setting up your PHP development environment is a straightforward process that will have you writing and testing your code in no time. Whether you're a beginner or just need a refresher, this guide will walk you through the steps to set up a productive PHP development environment.
Why Set Up a Local Development Environment?
Benefits of Local Development
Developing PHP locally offers a ton of advantages. You can code and test without affecting live websites, which helps prevent potential disasters. Plus, local development is faster since you're not dealing with the internet's latency. It’s a safe playground to experiment with new features and learn without pressure.
Key Components of a PHP Environment
To set up a local PHP development environment, you'll need a few key components:
- PHP: The language itself.
- Web Server: Usually Apache or Nginx.
- Database: Often MySQL or MariaDB.
- Development Tools: Code editor, version control, etc.
Let’s dive into getting each of these set up.
Installing PHP, Apache, and MySQL with XAMPP
What is XAMPP?
XAMPP is a free and open-source cross-platform web server solution stack package developed by Apache Friends. It provides an easy way to install Apache, MySQL, PHP, and Perl on your local machine.
Installing XAMPP
- Download XAMPP: Go to the official XAMPP website and download the version suitable for your operating system.
- Run the Installer: Follow the prompts to install XAMPP. You can usually go with the default settings.
- Start the Services: Open the XAMPP Control Panel and start the Apache and MySQL services.
Testing Your Installation
Once XAMPP is installed and running, you can test your PHP setup:
- Open your browser and go to
http://localhost/
. - Create a PHP file in the
htdocs
directory. For example,htdocs/test.php
. - Write a simple PHP script:
<?php echo "Hello, World!"; ?>
- Run the script: Open your browser and go to
http://localhost/test.php
. You should see "Hello, World!" displayed.
Example Code Execution
<?php
echo "Hello, World!";
?>
Result:
Hello, World!
Setting Up a Code Editor
Choosing the Right Editor
Your choice of code editor can significantly impact your productivity. Popular options include Visual Studio Code (VS Code), Sublime Text, and PHPStorm. Each has its own set of features and plugins to enhance PHP development.
Installing VS Code
- Download VS Code: Head to the VS Code website and download the installer.
- Install the Editor: Follow the prompts to complete the installation.
- Add PHP Extensions: In VS Code, go to the Extensions marketplace (the square icon in the sidebar) and install the PHP Intelephense extension for better syntax highlighting and IntelliSense.
Version Control with Git
Why Use Version Control?
Version control systems like Git keep track of changes to your codebase. This is crucial for collaboration, backup, and managing different versions of your project.
Setting Up Git
- Install Git: Download and install Git from the official site.
- Configure Git: Open your terminal or Git Bash and set up your user name and email:
git config --global user.name "Your Name" git config --global user.email "[email protected]"
- Initialize a Repository: Navigate to your project directory and run:
git init
- Add and Commit Files: Add your files to the repository and commit them:
git add . git commit -m "Initial commit"
Using Composer for Dependency Management
What is Composer?
Composer is a tool for managing dependencies in PHP. It allows you to declare the libraries your project depends on and installs them for you.
Installing Composer
- Download Composer: Visit the Composer website and follow the installation instructions for your operating system.
- Install Dependencies: Create a
composer.json
file in your project directory and specify your dependencies. For example:{ "require": { "monolog/monolog": "^2.0" } }
- Run Composer: In your terminal, navigate to your project directory and run:
composer install
Running Your PHP Project
Setting Up Virtual Hosts
Virtual hosts allow you to run multiple sites on your local machine using different domain names. This makes managing multiple projects easier.
Configuring Apache for Virtual Hosts
- Open Apache Configuration File: Locate the
httpd-vhosts.conf
file in your XAMPP installation directory (usually inapache/conf/extra
). - Add Virtual Host Entries: Add entries for each of your projects. For example:
<VirtualHost *:80> ServerAdmin [email protected] DocumentRoot "C:/xampp/htdocs/your_project" ServerName your_project.local ErrorLog "logs/your_project.local-error.log" CustomLog "logs/your_project.local-access.log" common </VirtualHost>
- Update Hosts File: Add entries to your
hosts
file to map the domain names tolocalhost
. For example:127.0.0.1 your_project.local
Accessing Your Project
Once configured, you can access your project by navigating to http://your_project.local
in your browser.
Conclusion
Setting up a PHP development environment might seem overwhelming at first, but breaking it down into manageable steps makes it much easier. With tools like XAMPP, VS Code, Git, and Composer, you can create a powerful and efficient workflow that lets you focus on coding rather than configuration. Happy coding!
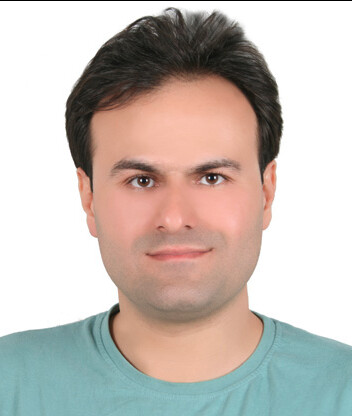
Sami Rahimi
Innovate relentlessly. Shape the future..
"Great job, keep these coming!" for more information visit us on <a href = https://www.fusiontechnologysolutions.in/php-course/>PHP Course</a>