Arrays in PHP: Indexed, Associative, and Multidimensional
When you're working with data in PHP, arrays are a go-to structure because they're versatile and easy to use. Whether you're dealing with a simple list of numbers or a complex set of data, arrays can handle it. Today, we’re diving into the three main types of arrays in PHP: indexed arrays, associative arrays, and multidimensional arrays. We’ll go over what they are, how to use them, and show some examples to make everything clear.
Indexed Arrays
What Are Indexed Arrays?
Indexed arrays use numeric indexes to store and access values. Think of it as a list where each item has a specific position, starting from zero. They’re perfect for ordered collections of data like a list of names or numbers.
How to Create Indexed Arrays
Creating an indexed array is straightforward. You can use the array()
function or the shorter []
syntax. Here’s an example:
$fruits = array("Apple", "Banana", "Cherry");
$vegetables = ["Carrot", "Pea", "Broccoli"];
Accessing Values in Indexed Arrays
To access an element, use its index. Here’s how you get the first fruit and the second vegetable:
echo $fruits[0]; // Outputs: Apple
echo $vegetables[1]; // Outputs: Pea
Adding and Removing Elements
Adding and removing elements in indexed arrays is easy. You can use array_push()
to add an element and array_pop()
to remove the last one:
array_push($fruits, "Orange");
echo implode(", ", $fruits); // Outputs: Apple, Banana, Cherry, Orange
array_pop($vegetables);
echo implode(", ", $vegetables); // Outputs: Carrot, Pea
Associative Arrays
What Are Associative Arrays?
Associative arrays use named keys instead of numeric indexes. This is great for when you want to associate values with specific identifiers, like storing user information where each piece of data has a clear label.
How to Create Associative Arrays
Here’s how you can create an associative array:
$user = array("name" => "John", "age" => 30, "email" => "[email protected]");
$car = ["brand" => "Toyota", "model" => "Corolla", "year" => 2020];
Accessing Values in Associative Arrays
To get the values, use the named keys:
echo $user["name"]; // Outputs: John
echo $car["model"]; // Outputs: Corolla
Adding and Removing Elements
Adding a new key-value pair or removing one is just as simple:
$user["location"] = "New York";
echo $user["location"]; // Outputs: New York
unset($car["year"]);
echo json_encode($car); // Outputs: {"brand":"Toyota","model":"Corolla"}
Multidimensional Arrays
What Are Multidimensional Arrays?
Multidimensional arrays are arrays within arrays. They’re useful for storing complex data, like a table or a grid. Each element in a multidimensional array can itself be an array.
How to Create Multidimensional Arrays
Here’s how you can set up a 2D array:
$matrix = array(
array(1, 2, 3),
array(4, 5, 6),
array(7, 8, 9)
);
Accessing Values in Multidimensional Arrays
To access a value, use multiple indices:
echo $matrix[0][1]; // Outputs: 2
echo $matrix[2][0]; // Outputs: 7
Adding and Removing Elements
You can also add and remove elements in multidimensional arrays. Here’s how you add a new row and remove an element:
$matrix[] = array(10, 11, 12);
print_r($matrix);
/*
Outputs:
Array
(
[0] => Array
(
[0] => 1
[1] => 2
[2] => 3
)
[1] => Array
(
[0] => 4
[1] => 5
[2] => 6
)
[2] => Array
(
[0] => 7
[1] => 8
[2] => 9
)
[3] => Array
(
[0] => 10
[1] => 11
[2] => 12
)
)
*/
unset($matrix[1][2]);
print_r($matrix);
/*
Outputs:
Array
(
[0] => Array
(
[0] => 1
[1] => 2
[2] => 3
)
[1] => Array
(
[0] => 4
[1] => 5
[2] =>
)
[2] => Array
(
[0] => 7
[1] => 8
[2] => 9
)
[3] => Array
(
[0] => 10
[1] => 11
[2] => 12
)
)
*/
Conclusion
Arrays in PHP are a powerful tool for managing data. Whether you're working with a simple list, a key-value pair collection, or a complex structure, PHP arrays have you covered. Indexed arrays are great for ordered lists, associative arrays make it easy to use named keys, and multidimensional arrays can handle complex data structures. Try these examples in your code to get a better feel for how they work and see how they can make your PHP coding more efficient and effective. Happy coding!
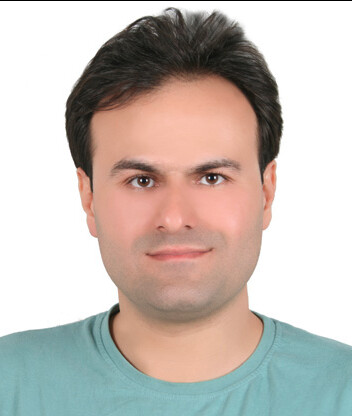
Sami Rahimi
Innovate relentlessly. Shape the future..
Recent Comments