Constants in PHP: A Guide to Using and Understanding Them
Hey there! In PHP, constants play a crucial role in keeping your code organized and secure. Let's dive into what constants are, how to use them effectively, and some best practices.
What are Constants?
Constants in PHP are like containers that hold values you don't expect to change during your script's execution. Once set, they remain fixed throughout the script. This makes them ideal for storing configuration settings, API keys, or any other data that shouldn't be altered accidentally.
Here’s how you define a constant:
<?php
define('SITE_NAME', 'My Website');
echo SITE_NAME; // Outputs: My Website
?>
Naming Conventions and Rules
When naming constants, it's common practice to use uppercase letters with underscores separating words (e.g., SITE_NAME
). Unlike variables, constants are case-sensitive by default in PHP.
Benefits of Using Constants
Constants offer several advantages:
- Readability: They make your code more readable by giving meaningful names to values.
- Security: Constants protect sensitive information like database credentials by preventing accidental changes.
- Global Scope: They are accessible from anywhere within the script, making them handy for configuration settings.
Constants vs. Variables
Unlike variables, constants cannot be changed or reassigned once defined. This immutability ensures that critical values remain consistent throughout your application’s lifecycle.
<?php
define('DB_USERNAME', 'admin');
echo DB_USERNAME; // Outputs: admin
// Attempting to change a constant (this will cause an error)
// DB_USERNAME = 'new_admin';
?>
Practical Use Cases
Consider using constants for:
- Configuration Settings:
define('DEBUG_MODE', true);
- API Keys:
define('API_KEY', 'your_api_key');
- Error Messages:
define('ERROR_MESSAGE', 'Oops! Something went wrong.');
Best Practices
To maintain clean and efficient code:
- Define constants at the top of your scripts to ensure they are set before being used.
- Document your constants using comments to explain their purpose and usage.
- Use meaningful names that describe the constant’s role or value.
Conclusion
In PHP, constants are invaluable for maintaining clarity, security, and efficiency in your codebase. By embracing constants, you enhance your ability to manage and protect crucial data throughout your application.
Now that you’ve grasped the basics of constants, why not try implementing them in your next PHP project? They’re a simple yet powerful tool that can make your coding life a whole lot easier.
Happy coding!
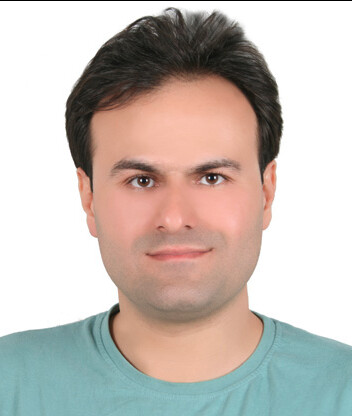
Sami Rahimi
Innovate relentlessly. Shape the future..
Recent Comments