Form Validation in PHP
Form validation is a crucial aspect of web development that ensures the data submitted by users is clean, accurate, and safe. In this blog post, we'll dive into the nuts and bolts of form validation in PHP, covering everything from the basics to more advanced techniques. We'll walk through real code examples, so you can see how it all works in action.
Why is Form Validation Important?
When users submit forms on your website, they might make mistakes or, worse, try to inject malicious code. Form validation helps prevent these issues by checking and sanitizing user input before processing it. This not only keeps your database safe but also improves user experience by catching errors early on.
Types of Form Validation
There are two main types of form validation: client-side and server-side.
Client-side validation is done in the user's browser, usually with JavaScript. It provides quick feedback but shouldn't be solely relied upon because users can bypass it.
Server-side validation happens on your server after the form data is submitted. This is where PHP comes into play. Server-side validation is essential for security and data integrity.
Setting Up a Simple Form in PHP
Let's start with a basic HTML form and a PHP script to handle the data.
HTML Form
Here’s a simple HTML form that collects a user's name and email:
<!DOCTYPE html>
<html>
<head>
<title>PHP Form Validation</title>
</head>
<body>
<form method="post" action="process_form.php">
Name: <input type="text" name="name"><br>
Email: <input type="text" name="email"><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
Basic PHP Validation
Now, let's create process_form.php
to handle the form submission and validate the input.
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = trim($_POST["name"]);
$email = trim($_POST["email"]);
$errors = [];
// Validate name
if (empty($name)) {
$errors[] = "Name is required.";
}
// Validate email
if (empty($email)) {
$errors[] = "Email is required.";
} elseif (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
$errors[] = "Invalid email format.";
}
// Display errors or success message
if (!empty($errors)) {
foreach ($errors as $error) {
echo "<p style='color:red;'>$error</p>";
}
} else {
echo "<p style='color:green;'>Form submitted successfully!</p>";
}
}
?>
In this script, we first check if the form was submitted using $_SERVER["REQUEST_METHOD"]
. We then trim the input values to remove extra spaces and initialize an array to hold any error messages. The filter_var
function is used to validate the email format.
Advanced Validation Techniques
Sanitizing User Input
Sanitizing input means cleaning it to remove any harmful characters. This helps prevent security issues like SQL injection and XSS attacks. Here's how you can sanitize the input:
$name = htmlspecialchars(strip_tags($name));
$email = filter_var($email, FILTER_SANITIZE_EMAIL);
In this example, htmlspecialchars
converts special characters to HTML entities, and strip_tags
removes HTML and PHP tags from the input. The FILTER_SANITIZE_EMAIL
filter removes all illegal characters from an email address.
Creating a Reusable Validation Function
To keep your code clean and DRY (Don't Repeat Yourself), you can create a reusable function for validation.
function validate_input($data, $type) {
$data = trim($data);
$data = strip_tags($data);
$data = htmlspecialchars($data);
if ($type == "email") {
if (!filter_var($data, FILTER_VALIDATE_EMAIL)) {
return "Invalid email format.";
}
} elseif ($type == "name") {
if (empty($data)) {
return "Name is required.";
}
}
return "";
}
// Usage
$name_error = validate_input($name, "name");
$email_error = validate_input($email, "email");
if ($name_error) {
echo "<p style='color:red;'>$name_error</p>";
}
if ($email_error) {
echo "<p style='color:red;'>$email_error</p>";
}
if (!$name_error && !$email_error) {
echo "<p style='color:green;'>Form submitted successfully!</p>";
}
This function takes the input data and the type of validation needed. It sanitizes the data and checks for errors based on the type specified.
Displaying Form Validation Errors
For a better user experience, display validation errors next to the form fields. This way, users can immediately see what they need to fix.
Enhanced HTML Form
Modify the HTML form to display errors:
<form method="post" action="process_form.php">
Name: <input type="text" name="name" value="<?php echo htmlspecialchars($name); ?>"><br>
<?php if ($name_error) echo "<p style='color:red;'>$name_error</p>"; ?>
Email: <input type="text" name="email" value="<?php echo htmlspecialchars($email); ?>"><br>
<?php if ($email_error) echo "<p style='color:red;'>$email_error</p>"; ?>
<input type="submit" value="Submit">
</form>
Here, we use PHP to pre-fill the form fields with the submitted values and display errors next to them. This approach provides immediate feedback and improves the form's usability.
Conclusion
Form validation in PHP is a vital skill for any web developer. By understanding and implementing both basic and advanced techniques, you can create secure, user-friendly forms. Remember to always sanitize and validate user input on the server side, even if you have client-side validation in place. Happy coding!
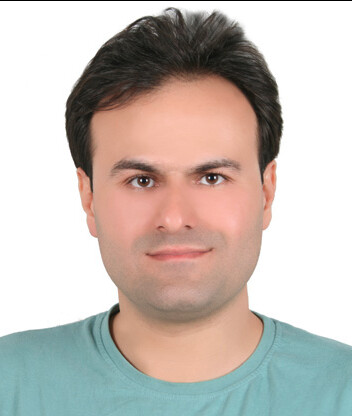
Sami Rahimi
Innovate relentlessly. Shape the future..
Recent Comments