PHP Strings: Functions and Manipulation
When it comes to PHP, handling strings is one of the most common tasks. Whether you’re building a simple website or a complex application, understanding how to manipulate strings can save you a lot of time and effort. In this post, we'll dive into some of the most useful PHP string functions and show you how to use them with plenty of examples.
String Length: strlen()
One of the basics you'll often need is finding out how long a string is. PHP makes this easy with the strlen()
function. This function takes a string as a parameter and returns the number of characters in the string, including spaces.
Example:
$string = "Hello, World!";
$length = strlen($string);
echo "The length of the string is: " . $length;
Output:
The length of the string is: 13
This is super helpful when you need to validate input, like ensuring a password is long enough or a username is within a certain character limit.
String Position: strpos()
If you need to find the position of a specific substring within a string, strpos()
is the function to use. It returns the position of the first occurrence of a substring.
Example:
$string = "Hello, World!";
$position = strpos($string, "World");
echo "The position of 'World' in the string is: " . $position;
Output:
The position of 'World' in the string is: 7
This is handy for checking if a string contains a certain word or phrase.
Replacing Text: str_replace()
Sometimes you need to replace parts of a string with something else. str_replace()
lets you do just that. It takes three parameters: the search string, the replacement string, and the original string.
Example:
$string = "Hello, World!";
$newString = str_replace("World", "PHP", $string);
echo $newString;
Output:
Hello, PHP!
This function is great for templating and modifying user input.
String to Upper and Lower Case: strtoupper() and strtolower()
Changing the case of a string can be useful, especially for formatting or ensuring consistency. strtoupper()
converts a string to uppercase, while strtolower()
converts it to lowercase.
Example:
$string = "Hello, World!";
$upper = strtoupper($string);
$lower = strtolower($string);
echo "Uppercase: " . $upper . "\n";
echo "Lowercase: " . $lower;
Output:
Uppercase: HELLO, WORLD!
Lowercase: hello, world!
These functions are perfect for formatting text to meet specific design requirements.
String Substring: substr()
Sometimes you need just a part of a string. The substr()
function allows you to extract a substring from a string, starting from a specified position.
Example:
$string = "Hello, World!";
$substring = substr($string, 7, 5);
echo $substring;
Output:
World
This is useful for truncating text, such as creating previews or summaries.
Removing Whitespace: trim()
When dealing with user input, you often need to remove unwanted whitespace from the beginning and end of strings. The trim()
function handles this neatly.
Example:
$string = " Hello, World! ";
$trimmed = trim($string);
echo "Before: '" . $string . "'\n";
echo "After: '" . $trimmed . "'";
Output:
Before: ' Hello, World! '
After: 'Hello, World!'
This is crucial for cleaning up user input before processing or storing it.
Splitting Strings: explode()
Breaking a string into an array based on a delimiter is easy with the explode()
function. This is especially useful for parsing data.
Example:
$string = "apple,banana,orange";
$array = explode(",", $string);
print_r($array);
Output:
Array
(
[0] => apple
[1] => banana
[2] => orange
)
Use this function to handle CSV data or to break up a sentence into words.
Joining Strings: implode()
The opposite of explode()
, the implode()
function joins array elements into a single string with a specified delimiter.
Example:
$array = ["apple", "banana", "orange"];
$string = implode(", ", $array);
echo $string;
Output:
apple, banana, orange
This function is perfect for generating lists or combining elements into a single string for output or storage.
Conclusion
Mastering PHP string functions can greatly simplify your coding tasks. From basic operations like finding string lengths to more complex manipulations like replacing text and splitting strings, these functions are essential tools in your PHP arsenal. By understanding and using these functions, you'll be able to handle strings efficiently and effectively in your projects. Happy coding!
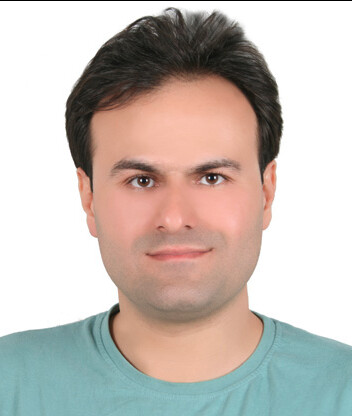
Sami Rahimi
Innovate relentlessly. Shape the future..
Recent Comments