Looping in PHP: For, While, and Do-While
Loops are a fundamental part of any programming language, and PHP is no exception. They let you execute a block of code multiple times, which is incredibly useful for tasks like processing arrays or performing repetitive calculations. In this post, we'll explore the three main types of loops in PHP: for
, while
, and do-while
. We'll go over their syntax, usage, and some examples to help you get a good grasp on how to use them effectively.
Understanding For Loops
A for
loop is great when you know in advance how many times you want to execute a block of code. It consists of three parts: the initialization, the condition, and the increment/decrement. This structure makes for
loops perfect for iterating over arrays or running a loop a specific number of times.
Syntax of For Loops
Here's the basic syntax of a for
loop:
for (initialization; condition; increment) {
// code to be executed
}
The initialization
happens once at the beginning, the condition
is checked before each iteration, and the increment
is executed at the end of each iteration.
Example of a For Loop
Let's see a for
loop in action with a simple example that prints numbers from 1 to 5.
for ($i = 1; $i <= 5; $i++) {
echo $i . " ";
}
Output:
1 2 3 4 5
In this example, $i
is initialized to 1, the loop runs as long as $i
is less than or equal to 5, and $i
is incremented by 1 after each iteration.
Exploring While Loops
A while
loop is useful when you want to repeat a block of code as long as a specific condition is true. Unlike for
loops, while
loops are more flexible because the condition is evaluated before each iteration, and you don't need to know in advance how many times the loop will run.
Syntax of While Loops
Here's the basic syntax of a while
loop:
while (condition) {
// code to be executed
}
The condition
is checked before each iteration. If it's true, the code inside the loop runs. If it's false, the loop stops.
Example of a While Loop
Let's look at an example where we use a while
loop to print numbers from 1 to 5.
$i = 1;
while ($i <= 5) {
echo $i . " ";
$i++;
}
Output:
1 2 3 4 5
In this example, the loop starts with $i
set to 1. The loop continues running as long as $i
is less than or equal to 5. Inside the loop, we print $i
and then increment it by 1.
Understanding Do-While Loops
A do-while
loop is similar to a while
loop, but with one key difference: the block of code runs at least once, even if the condition is false from the beginning. This is because the condition is checked after the code has executed.
Syntax of Do-While Loops
Here's the basic syntax of a do-while
loop:
do {
// code to be executed
} while (condition);
The code inside the do
block runs first, and then the condition
is evaluated. If it's true, the loop runs again. If it's false, the loop stops.
Example of a Do-While Loop
Let's see an example of a do-while
loop that prints numbers from 1 to 5.
$i = 1;
do {
echo $i . " ";
$i++;
} while ($i <= 5);
Output:
1 2 3 4 5
In this example, the code inside the do
block runs first with $i
set to 1. Then the condition $i <= 5
is checked. Since it's true, the loop continues. The process repeats until $i
is greater than 5.
Practical Tips for Using Loops in PHP
Avoid Infinite Loops
Be careful to ensure your loop conditions will eventually be met. Infinite loops can cause your program to freeze or crash. Always double-check your conditions and increments.
Break and Continue
Use break
to exit a loop early and continue
to skip the current iteration and move to the next one. These can be handy for optimizing your loops and handling special cases.
Nested Loops
You can nest loops inside each other for more complex scenarios, like iterating through multi-dimensional arrays. Just be mindful of the potential performance impact.
Example with Break and Continue
Here's an example using break
and continue
:
for ($i = 1; $i <= 10; $i++) {
if ($i == 5) {
continue; // Skip the number 5
}
if ($i == 8) {
break; // Stop the loop at 8
}
echo $i . " ";
}
Output:
1 2 3 4 6 7
In this example, the loop skips printing the number 5 and stops completely when it reaches 8.
Conclusion
Understanding and using loops in PHP is essential for writing efficient and effective code. Whether you're using for
, while
, or do-while
loops, they each have their strengths and best use cases. Practice with different scenarios to get a feel for which loop works best in different situations. Happy coding!
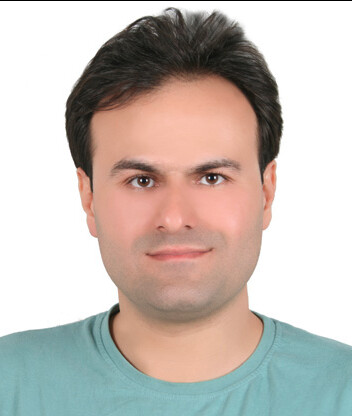
Sami Rahimi
Innovate relentlessly. Shape the future..
Recent Comments