Error Handling in PHP
Dealing with errors is a crucial part of developing any application. In PHP, error handling helps you catch and respond to errors in a controlled way, making your applications more robust and user-friendly. Let's dive into how you can handle errors in PHP, from basic error handling to advanced techniques.
Understanding PHP Errors
PHP errors can come in many forms, like syntax errors, runtime errors, and logical errors. Each of these needs to be handled differently to maintain a smooth user experience.
Types of PHP Errors
In PHP, there are several types of errors you might encounter:
- Parse Errors: These occur when there is a syntax mistake in your code.
- Fatal Errors: These happen when PHP encounters an error it can't recover from, such as calling a function that doesn't exist.
- Warning Errors: These are non-fatal errors that alert you to potential problems in your code, but the script continues to execute.
- Notice Errors: These are minor errors that indicate something that could potentially be problematic, like using an undefined variable.
Example of an Error
Here's a simple example of a PHP script with a deliberate syntax error:
<?php
echo "Hello, World!"
?>
Running this script will result in a parse error because the semicolon is missing after the echo statement:
Parse error: syntax error, unexpected '?>' in /path/to/your/script.php on line 2
Basic Error Handling
Handling errors in PHP can be as simple as using built-in error handling functions. This helps in displaying more user-friendly error messages and logging errors for debugging.
Using die()
and exit()
The die()
and exit()
functions are simple ways to handle errors. They stop the script and can display a message.
<?php
$file = fopen("nonexistentfile.txt", "r") or die("Unable to open file!");
?>
In this example, if the file doesn't exist, PHP will stop executing the script and display "Unable to open file!".
Custom Error Handling
For more flexibility, PHP allows you to create custom error handlers. This is useful for logging errors or displaying custom error messages.
Creating a Custom Error Handler
You can create a custom error handler by defining a function and using set_error_handler()
.
<?php
function customError($errno, $errstr) {
echo "<b>Error:</b> [$errno] $errstr<br>";
echo "Ending Script";
die();
}
set_error_handler("customError");
// Trigger an error
echo($test);
?>
In this example, the custom error handler will catch the undefined variable $test
and display a custom error message before stopping the script.
Example Output
When running the above code, you will see:
Error: [8] Undefined variable: test
Ending Script
Advanced Error Handling
For more complex applications, PHP provides more advanced error handling mechanisms like try-catch
blocks and exceptions.
Using Try-Catch Blocks
try-catch
blocks allow you to catch exceptions and handle them gracefully.
<?php
function divide($dividend, $divisor) {
if($divisor == 0) {
throw new Exception("Division by zero");
}
return $dividend / $divisor;
}
try {
echo divide(10, 0);
} catch(Exception $e) {
echo "Caught exception: " . $e->getMessage();
}
?>
Example Output
When running the code with a division by zero, the output will be:
Caught exception: Division by zero
Logging Errors
Logging errors to a file or a logging system is crucial for debugging and maintaining a healthy application.
Enabling Error Logging
You can configure PHP to log errors by setting error_log
in your php.ini
file.
log_errors = On
error_log = /path/to/your/error.log
Example of Writing to a Log
Here's how you can log errors manually in your code:
<?php
error_log("This is an error message!", 3, "/path/to/your/error.log");
?>
This will write "This is an error message!" to the specified log file.
Conclusion
Handling errors in PHP effectively is key to building reliable and user-friendly applications. Whether you're using simple error handling functions, custom error handlers, or advanced techniques like try-catch
blocks, understanding and implementing proper error handling will save you time and headaches. Remember to log your errors for easier debugging and to keep your error messages user-friendly. Happy coding!
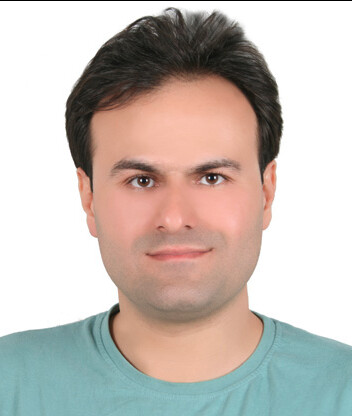
Sami Rahimi
Innovate relentlessly. Shape the future..
Recent Comments