Introduction to PHP
Hey there! Welcome to our crash course on PHP (Hypertext Preprocessor). Whether you're curious about diving into web development or looking to expand your coding toolkit, PHP is a fantastic language to start with. In this guide, we'll walk you through the basics and get you up and running with some practical examples.
What is PHP?
PHP is a server-side scripting language primarily designed for web development. It's versatile and used by millions of websites to handle tasks like generating dynamic content, processing forms, managing databases, and more. Unlike HTML, which is static, PHP enables websites to interact with users and customize responses based on input.
Why PHP?
PHP's popularity stems from its ease of use and integration capabilities with various databases like MySQL, PostgreSQL, and others. It's open-source, actively maintained, and has a vast community contributing to its ecosystem. These qualities make it a robust choice for both beginners and seasoned developers alike.
<?php
echo "Hello, PHP!";
?>
Output:
Hello, PHP!
Getting Started with PHP
To start writing PHP, all you need is a text editor and a web server with PHP installed. If you're just beginning, tools like XAMPP or MAMP provide everything in a single package, including Apache (the web server), MySQL (database), and PHP itself.
Writing Your First PHP Script
Let's create a simple PHP script to display a message:
<?php
$message = "Hello, PHP!";
echo $message;
?>
Output:
Hello, PHP!
Variables and Data Types in PHP
In PHP, variables are used to store data. Unlike some languages, PHP does not require explicit declaration of variable types. Here are a few examples of variable usage:
Example 1: String Variable
<?php
$name = "Alice";
echo "Hello, $name!";
?>
Output:
Hello, Alice!
Example 2: Numeric Variables
<?php
$number1 = 10;
$number2 = 5;
$sum = $number1 + $number2;
echo "The sum of $number1 and $number2 is: $sum";
?>
Output:
The sum of 10 and 5 is: 15
Control Structures: Conditionals and Loops
PHP supports typical control structures like if-else statements and loops. These are fundamental for creating dynamic web applications.
Example: Using if-else Statement
<?php
$grade = 85;
if ($grade >= 60) {
echo "Passed!";
} else {
echo "Failed!";
}
?>
Output:
Passed!
Example: Using a for Loop
<?php
for ($i = 1; $i <= 5; $i++) {
echo "Count: $i<br>";
}
?>
Output:
Count: 1
Count: 2
Count: 3
Count: 4
Count: 5
Conclusion
PHP provides a solid foundation for building dynamic web applications. From handling user input to interacting with databases, its versatility makes it a go-to language for web developers worldwide. As you continue your journey with PHP, explore its extensive documentation and community resources to expand your skills further.
Ready to dive deeper? Stay tuned for more advanced PHP tutorials coming your way soon! Happy coding!
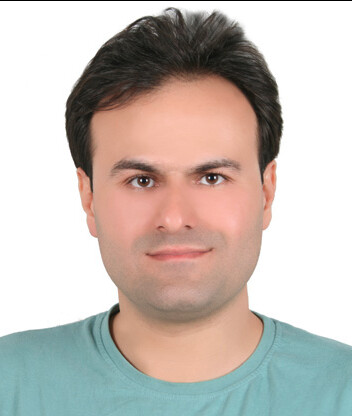
Sami Rahimi
Innovate relentlessly. Shape the future..
Recent Comments