Exploring Superglobals in PHP
PHP, being a versatile language for web development, offers a set of predefined variables called superglobals that are accessible from any scope in your scripts. These variables play a crucial role in handling forms, cookies, sessions, and server details effortlessly.
What are Superglobals?
Superglobals are PHP's built-in variables that are always accessible, regardless of scope. They provide essential information about the environment and user input. Let's dive into some of the most commonly used ones:
$_GET and $_POST
$_GET is used to collect data sent in the URL parameters, typically from an HTML form using the GET method. It's handy for retrieving data when the information doesn't need to be secured, such as search queries.
// Example of $_GET usage
// Assuming URL is like: example.php?name=John&age=30
$name = $_GET['name'];
$age = $_GET['age'];
echo "Name: $name, Age: $age";
// Output: Name: John, Age: 30
$_POST, on the other hand, retrieves data sent through the HTTP POST method, usually from forms where sensitive information like passwords should be sent securely.
// Example of $_POST usage
$name = $_POST['name'];
$email = $_POST['email'];
echo "Name: $name, Email: $email";
$_SESSION
$_SESSION is used to store session variables that are accessible across multiple pages. It's invaluable for maintaining user login sessions and persisting data during a user's visit to your site.
// Example of $_SESSION usage
session_start();
$_SESSION['username'] = 'john_doe';
$_SESSION['email'] = '[email protected]';
echo "Session variables are set.";
$_COOKIE
$_COOKIE holds all cookies sent by the client to the server. Cookies are useful for remembering user preferences or login details.
// Example of $_COOKIE usage
$cookie_name = "user";
$cookie_value = "John Doe";
setcookie($cookie_name, $cookie_value, time() + (86400 * 30), "/"); // 30 days
echo "Cookie '$cookie_name' is set.";
$_SERVER
$_SERVER contains information about headers, paths, and script locations. It's particularly useful for obtaining details about the server environment.
// Example of $_SERVER usage
echo 'Server IP Address: ' . $_SERVER['SERVER_ADDR'];
echo 'User Agent: ' . $_SERVER['HTTP_USER_AGENT'];
$_FILES
$_FILES holds information about uploaded files via HTTP POST. It's essential for handling file uploads securely.
// Example of $_FILES usage
echo "Uploaded file name: " . $_FILES['file']['name'];
echo "File size: " . $_FILES['file']['size'];
Best Practices for Using Superglobals
-
Sanitize User Input: Always sanitize data from $_GET, $_POST, and $_COOKIE to prevent security vulnerabilities like SQL injection and XSS attacks.
-
Use isset() or empty(): Before accessing any superglobal variable directly, ensure it's set to avoid PHP notices.
-
Avoid Global Scope: Minimize the use of superglobals in the global scope to enhance code maintainability and readability.
Superglobals in PHP streamline common web development tasks by providing access to essential data and server information. Understanding how to leverage them securely is fundamental to building robust and efficient web applications.
Next time you're handling form submissions or managing user sessions in PHP, remember these super handy superglobals!
Happy coding! 🚀
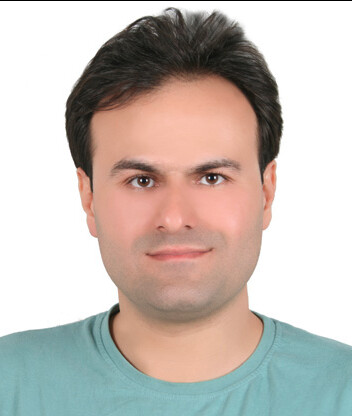
Sami Rahimi
Innovate relentlessly. Shape the future..
Recent Comments