PHP Syntax and Basic Constructs
Hey there! If you're diving into the world of web development, PHP is a fantastic language to learn. It's widely used, easy to pick up, and powers a huge chunk of the web. In this post, we'll walk through the basics of PHP syntax and its fundamental constructs. By the end, you’ll be ready to start coding your own PHP scripts. Let's get started!
What is PHP?
PHP, which stands for "PHP: Hypertext Preprocessor," is a popular server-side scripting language. It's designed specifically for web development but can be used as a general-purpose programming language. The beauty of PHP lies in its ability to be embedded into HTML, making it a powerful tool for creating dynamic web pages.
<?php
echo "Hello, World!";
?>
When you run this code on a server, it will display:
Hello, World!
PHP Syntax Basics
Opening and Closing Tags
PHP code is enclosed in <?php
and ?>
tags. These tags tell the server to process the enclosed code as PHP.
<?php
// PHP code goes here
?>
Any code outside these tags is treated as HTML. This makes PHP great for mixing with HTML to create dynamic content.
Comments
Comments are essential for making your code understandable. PHP supports both single-line and multi-line comments.
<?php
// This is a single-line comment
# This is another single-line comment
/*
This is a
multi-line comment
*/
?>
Variables
Variables in PHP start with a $
sign, followed by the variable name. PHP is a loosely typed language, so you don’t need to declare the type of variable.
<?php
$name = "John";
$age = 30;
echo "Name: " . $name . ", Age: " . $age;
?>
This script will output:
Name: John, Age: 30
PHP Data Types
PHP supports several data types including integers, floats, strings, arrays, objects, and more. Let's look at a few of them.
Strings
Strings are sequences of characters, like "Hello, World!".
<?php
$text = "Hello, World!";
echo $text;
?>
You'll see:
Hello, World!
Integers and Floats
Integers are whole numbers, while floats (or doubles) are numbers with decimal points.
<?php
$int = 42;
$float = 3.14;
echo "Integer: " . $int . ", Float: " . $float;
?>
This will print:
Integer: 42, Float: 3.14
Arrays
Arrays store multiple values in one variable. PHP supports both indexed and associative arrays.
<?php
$fruits = array("Apple", "Banana", "Cherry");
echo "I like " . $fruits[1];
?>
The output will be:
I like Banana
Control Structures
Control structures let you control the flow of your script.
If-Else Statements
If-else statements execute code based on conditions.
<?php
$hour = 10;
if ($hour < 12) {
echo "Good morning!";
} else {
echo "Good afternoon!";
}
?>
Since $hour
is 10, the script will display:
Good morning!
Switch Statements
Switch statements are great for comparing one variable against many values.
<?php
$color = "red";
switch ($color) {
case "blue":
echo "Color is blue";
break;
case "red":
echo "Color is red";
break;
default:
echo "Color is neither blue nor red";
}
?>
This outputs:
Color is red
Loops
Loops are used for executing a block of code repeatedly.
While Loop
The while
loop executes as long as a condition is true.
<?php
$i = 1;
while ($i <= 5) {
echo "Number: " . $i . "<br>";
$i++;
}
?>
This will print:
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
For Loop
The for
loop is ideal for iterating a specific number of times.
<?php
for ($i = 1; $i <= 5; $i++) {
echo "Number: " . $i . "<br>";
}
?>
And you'll see the same result as above.
Functions
Functions let you encapsulate code into reusable blocks.
<?php
function greet($name) {
return "Hello, " . $name;
}
echo greet("Alice");
?>
This will display:
Hello, Alice
Conclusion
Learning PHP opens up a lot of possibilities for building dynamic and interactive web applications. We’ve covered the basics of PHP syntax and constructs, but this is just the beginning. There’s so much more to explore, like working with databases, handling forms, and using PHP frameworks. Keep practicing, and soon you'll be building amazing things with PHP!
Happy coding!
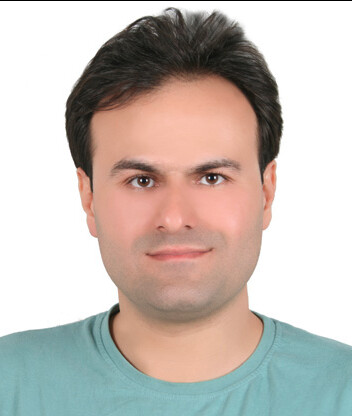
Sami Rahimi
Innovate relentlessly. Shape the future..
Recent Comments