Understanding PHP PDO: The Modern Way to Handle Databases in PHP
If you've been coding with PHP for a while, you’ve likely worked with databases. In the old days, developers used MySQL functions directly, but there's a better way now: PDO, or PHP Data Objects. Let's dive into what PDO is and how to use it to make your database interactions more secure and efficient.
What is PHP PDO?
PDO stands for PHP Data Objects. It’s a database access layer providing a uniform method of access to multiple databases. That means with PDO, you can switch your database driver (e.g., from MySQL to PostgreSQL) with minimal changes to your code. PDO also helps protect your application from SQL injection attacks, making your code more secure.
Why Use PDO?
PDO offers several advantages over older PHP database extensions:
- Database Agnostic: Easily switch between databases.
- Prepared Statements: Protect against SQL injection.
- Error Handling: Better error management with exceptions.
- Flexible Fetch Modes: Various ways to fetch data from queries.
Getting Started with PHP PDO
To get started with PDO, you first need to create a PDO instance by connecting to your database. Below is a simple example of how to connect to a MySQL database using PDO.
Connecting to a Database
<?php
$dsn = 'mysql:host=localhost;dbname=testdb';
$username = 'root';
$password = '';
try {
$pdo = new PDO($dsn, $username, $password);
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "Connected successfully";
} catch (PDOException $e) {
echo 'Connection failed: ' . $e->getMessage();
}
?>
In this example, dsn
stands for Data Source Name, which includes the type of database, host, and database name. The setAttribute
method sets the error mode to throw exceptions, making error handling much easier.
Executing SQL Queries
Once connected, you can execute SQL queries. Let's look at how to perform common database operations like selecting, inserting, updating, and deleting data.
Selecting Data
Fetching data from a database is straightforward with PDO. Here’s an example of how to retrieve data:
<?php
$sql = 'SELECT * FROM users';
$stmt = $pdo->query($sql);
while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) {
echo $row['username'] . "<br>";
}
?>
In this code, query
executes the SQL statement, and fetch
retrieves the rows. PDO::FETCH_ASSOC
makes the method return an associative array.
Using Prepared Statements
Prepared statements are a powerful feature in PDO, offering both security and performance benefits.
<?php
$sql = 'SELECT * FROM users WHERE email = :email';
$stmt = $pdo->prepare($sql);
$stmt->execute(['email' => '[email protected]']);
$user = $stmt->fetch(PDO::FETCH_ASSOC);
echo $user['username'];
?>
In this snippet, prepare
creates a prepared statement, and execute
runs it with the specified parameters. This approach prevents SQL injection by separating SQL logic from data.
Inserting Data
Inserting data with PDO is just as simple and benefits from prepared statements for security.
<?php
$sql = 'INSERT INTO users (username, email) VALUES (:username, :email)';
$stmt = $pdo->prepare($sql);
$stmt->execute(['username' => 'newuser', 'email' => '[email protected]']);
echo "New record created successfully";
?>
By using execute
with an associative array, you can easily insert user data into your database.
Updating Data
Updating records in your database is also easy with PDO.
<?php
$sql = 'UPDATE users SET email = :email WHERE username = :username';
$stmt = $pdo->prepare($sql);
$stmt->execute(['email' => '[email protected]', 'username' => 'newuser']);
echo "Record updated successfully";
?>
With this method, you can update specific records by specifying conditions in the SQL statement.
Deleting Data
Finally, deleting records is straightforward and secure with prepared statements.
<?php
$sql = 'DELETE FROM users WHERE username = :username';
$stmt = $pdo->prepare($sql);
$stmt->execute(['username' => 'newuser']);
echo "Record deleted successfully";
?>
This example shows how to remove a user from the database by their username.
Error Handling in PDO
PDO offers robust error handling mechanisms, which are essential for debugging and maintaining your code.
Using Exceptions
Setting PDO to throw exceptions on errors allows you to catch and handle them gracefully.
<?php
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
try {
$pdo->exec("INVALID SQL");
} catch (PDOException $e) {
echo 'Error: ' . $e->getMessage();
}
?>
With this setup, any SQL errors will be caught and displayed, preventing your script from crashing unexpectedly.
Conclusion
PHP PDO is a powerful tool for managing database interactions in a secure and flexible way. By using prepared statements, you can protect your application from SQL injection attacks and make your code database-agnostic. Whether you're selecting, inserting, updating, or deleting data, PDO makes these tasks straightforward and secure. Happy coding!
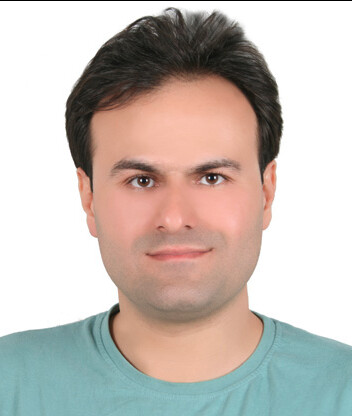
Sami Rahimi
Innovate relentlessly. Shape the future..
Recent Comments