A Comprehensive Guide to Laravel Facades
Laravel is one of the best PHP frameworks, known for its elegant syntax and the power of many features. Among all these powerhouse features, Laravel Facades have their own specialty when it comes to providing a unique and convenient way of accessing other services and values from the framework. This article will explain how to work with Laravel Facades, their benefits, and some good practices.
Understanding Facades
Facades in Laravel are static interfaces for classes in the application's service container and, therefore, act as a static proxy to the class resolved from the service container, in order to allow a generic way of referring to the methods of that class without actually instantiating the class.
How Facades Work
Behind the scenes, façades are nothing more than some simple PHP magic methods. When a method on a facade is called, that call is forwarded behind the scenes to the service container through the service provider bound to that particular facade. This is very easily manifested by the native base class of the Laravel Facade, which on its side ensures that the service container returns the right instance.
Commonly Used Facades
Laravel comes with a collection of pre-built Facades out of the box. Few Facades that we use more often are:
- DB: For database operations
- Cache: For caching operations
- Log: For logging messages
- Queue: For interacting with job queues
Benefits of Using Facades
Simplified Syntax
Facades offer a simple interface to the complex underlying services in the application. You will actually have a simple static method call instead of injection and binding dependencies.
Easy Access to Services
Facades provide an easy way to access several of Laravel's services without the need for application developers to inject those services into their classes.
Improved Code Readability and Maintenance
With Facades, your code can be more readable and maintainable. The static method calls can make the code cleaner and easier to understand at a glance.
Examples of Productivity Gains
Using Facades can speed up development by reducing boilerplate code. For example, accessing the cache is as simple as:
Cache::put('key', 'value', $minutes);
This ease of use can lead to significant productivity gains in everyday development tasks.
How to Create Custom Facades
Creating a custom Facade in Laravel involves a few steps:
-
Create the underlying class: This class contains the logic you want to expose via the Facade.
namespace App\Services; class MyService { public function performAction() { return "Action performed!"; } }
-
Register the service in the container: Add the service to Laravel's service container in a service provider.
// In AppServiceProvider or a dedicated service provider public function register() { $this->app->singleton(MyService::class, function ($app) { return new MyService(); }); }
-
Create the Facade class: This class extends Laravel's base Facade class and defines a method to get the service from the container.
namespace App\Facades; use Illuminate\Support\Facades\Facade; class MyServiceFacade extends Facade { protected static function getFacadeAccessor() { return \App\Services\MyService::class; } }
-
Register the Facade: Add the Facade to the
aliases
array inconfig/app.php
.'aliases' => [ 'MyService' => App\Facades\MyServiceFacade::class, ],
Now you can use your custom Facade in your application:
MyService::performAction();
Best Practices for Using Facades
When to Use and When to Avoid Using Facades
Facades are great for quick access to services, but they should be used judiciously. Over-reliance on Facades can make testing harder and increase coupling. Use Facades for services that are truly global in nature, but consider dependency injection for more complex or critical parts of your application.
Potential Pitfalls and How to Avoid Them
One of the pitfalls is testing the code that has heavy dependency on Facades. Laravel provides helper methods used for mocking the Facades during test pass. Additionally, one should mind performance issues because overuse of Facades could be the cause of overhead.
Performance Considerations
While Facades are convenient, they do add a small performance overhead due to the need to resolve services from the container. For performance-critical code, consider directly injecting dependencies.
Common Misconceptions and FAQs
Misconception: Facades Are Global Static Helpers
While Facades provide a static interface, they are not global static helpers. They are resolved from the service container, ensuring that they are testable and follow Laravel's dependency injection principles.
FAQ: How Do Facades Impact Testing?
Facades can actually make testing difficult, though, because hidden dependencies are tough to sniff out. Laravel offers many tools around facades — such as Facade::shouldReceive()
— that will let you effectively unit test code using facades.
Conclusion
The Laravel Facades provide a wonderful and powerful way to work with services under the hood of the Laravel application framework. Due to an understanding of how they operate and best practice, one can make good use of facades to write clean and maintainable, effectively efficient code. Be it using built-in implementations or custom facades, it dramatically improves your development experience in Laravel.
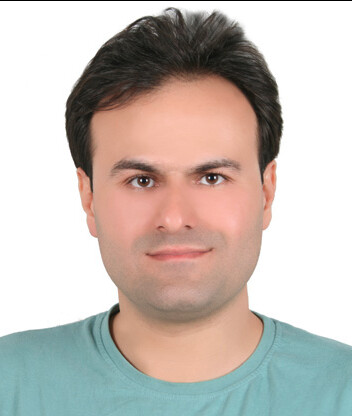
Sami Rahimi
Innovate relentlessly. Shape the future..
Recent Comments